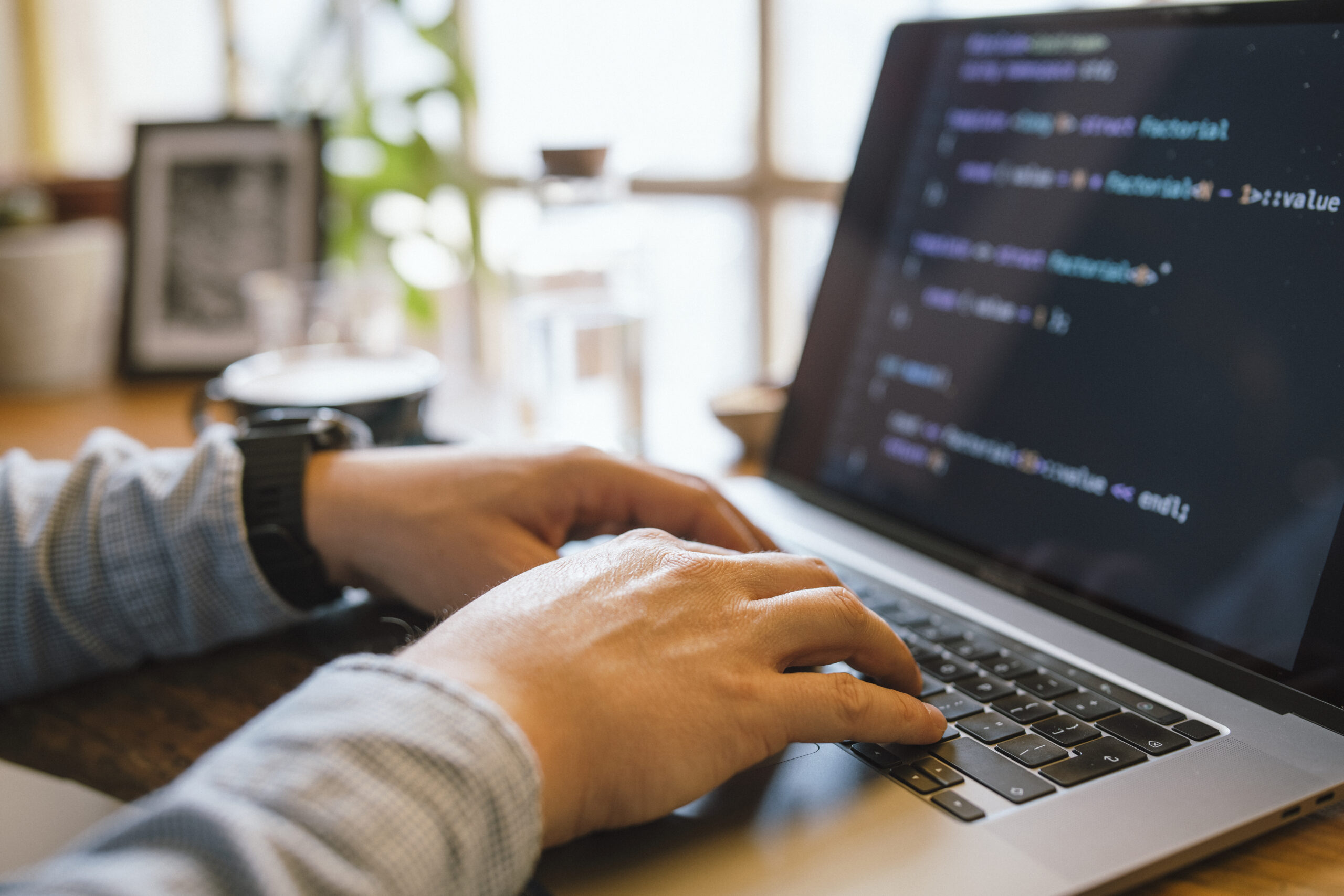
Debugging is one of the most important — nevertheless normally disregarded — techniques inside of a developer’s toolkit. It's not just about fixing broken code; it’s about comprehending how and why items go Mistaken, and Mastering to Assume methodically to unravel challenges competently. Whether you are a starter or simply a seasoned developer, sharpening your debugging competencies can help you save several hours of irritation and radically transform your efficiency. Here's various procedures that will help builders stage up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
One of the fastest strategies developers can elevate their debugging abilities is by mastering the tools they use everyday. When producing code is a single A part of development, recognizing the way to interact with it effectively all through execution is equally essential. Fashionable progress environments arrive equipped with strong debugging capabilities — but numerous builders only scratch the surface area of what these tools can perform.
Just take, as an example, an Integrated Progress Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools help you set breakpoints, inspect the value of variables at runtime, action via code line by line, as well as modify code over the fly. When applied properly, they Permit you to observe particularly how your code behaves throughout execution, which happens to be priceless for monitoring down elusive bugs.
Browser developer equipment, which include Chrome DevTools, are indispensable for front-close developers. They help you inspect the DOM, keep track of community requests, view real-time effectiveness metrics, and debug JavaScript in the browser. Mastering the console, sources, and community tabs can turn aggravating UI challenges into manageable duties.
For backend or process-level developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage in excess of functioning processes and memory management. Mastering these applications might have a steeper Finding out curve but pays off when debugging general performance issues, memory leaks, or segmentation faults.
Over and above your IDE or debugger, turn into snug with version Handle programs like Git to be familiar with code history, locate the exact second bugs have been launched, and isolate problematic improvements.
Finally, mastering your tools indicates going past default options and shortcuts — it’s about building an intimate understanding of your advancement natural environment to make sure that when challenges crop up, you’re not shed in the dark. The better you know your tools, the greater time you could spend solving the actual problem rather than fumbling through the procedure.
Reproduce the condition
One of the more significant — and infrequently neglected — methods in successful debugging is reproducing the issue. Prior to jumping into the code or earning guesses, builders need to have to make a steady atmosphere or state of affairs wherever the bug reliably appears. With out reproducibility, correcting a bug will become a match of opportunity, normally resulting in wasted time and fragile code variations.
Step one in reproducing an issue is accumulating as much context as possible. Check with queries like: What actions brought about the issue? Which ecosystem was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The more depth you've, the easier it gets to isolate the exact problems below which the bug takes place.
When you’ve gathered sufficient facts, attempt to recreate the situation in your local natural environment. This could indicate inputting exactly the same knowledge, simulating similar consumer interactions, or mimicking procedure states. If the issue seems intermittently, consider composing automatic tests that replicate the edge scenarios or point out transitions concerned. These assessments don't just support expose the problem but additionally protect against regressions in the future.
At times, The problem may be surroundings-distinct — it'd happen only on sure operating techniques, browsers, or underneath individual configurations. Using resources like virtual equipment, containerization (e.g., Docker), or cross-browser tests platforms is usually instrumental in replicating this kind of bugs.
Reproducing the trouble isn’t merely a action — it’s a mentality. It requires patience, observation, as well as a methodical technique. But when you finally can continually recreate the bug, you're currently halfway to fixing it. Having a reproducible situation, You can utilize your debugging equipment far more properly, take a look at probable fixes safely and securely, and converse far more Plainly using your crew or end users. It turns an abstract complaint into a concrete obstacle — Which’s wherever builders thrive.
Study and Comprehend the Error Messages
Mistake messages in many cases are the most worthy clues a developer has when a thing goes Mistaken. In lieu of observing them as aggravating interruptions, developers should master to take care of error messages as direct communications within the technique. They usually let you know exactly what transpired, the place it occurred, and sometimes even why it transpired — if you understand how to interpret them.
Begin by reading the concept very carefully As well as in entire. Several builders, particularly when under time tension, look at the initial line and immediately start out producing assumptions. But deeper from the error stack or logs may perhaps lie the real root trigger. Don’t just duplicate and paste error messages into search engines like google and yahoo — read through and comprehend them initially.
Break the mistake down into parts. Could it be a syntax mistake, a runtime exception, or possibly a logic mistake? Does it issue to a particular file and line range? What module or perform activated it? These concerns can information your investigation and point you toward the liable code.
It’s also useful to be aware of the terminology from the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java frequently observe predictable patterns, and Finding out to acknowledge these can significantly hasten your debugging process.
Some mistakes are obscure or generic, As well as in those situations, it’s important to examine the context by which the error happened. Look at associated log entries, input values, and up to date variations in the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger sized problems and provide hints about likely bugs.
In the long run, mistake messages are not your enemies—they’re your guides. Studying to interpret them appropriately turns chaos into clarity, serving to you pinpoint difficulties a lot quicker, reduce debugging time, and become a a lot more productive and self-confident developer.
Use Logging Correctly
Logging is Among the most highly effective applications inside of a developer’s debugging toolkit. When made use of effectively, it offers real-time insights into how an software behaves, aiding you realize what’s taking place beneath the hood with no need to pause execution or stage through the code line by line.
A great logging strategy starts with knowing what to log and at what amount. Popular logging degrees include things like DEBUG, Details, WARN, ERROR, and Lethal. Use DEBUG for in-depth diagnostic facts through growth, Data for here common activities (like effective start-ups), Alert for likely concerns that don’t break the applying, Mistake for real problems, and Lethal if the method can’t continue.
Prevent flooding your logs with abnormal or irrelevant information. Too much logging can obscure significant messages and slow down your system. Deal with essential occasions, point out adjustments, input/output values, and significant choice details within your code.
Structure your log messages Obviously and consistently. Include things like context, including timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what problems are met, and what branches of logic are executed—all with no halting This system. They’re Specifically important in creation environments where by stepping by means of code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
In the long run, sensible logging is about harmony and clarity. Which has a nicely-considered-out logging approach, you'll be able to lessen the time it takes to spot troubles, attain deeper visibility into your programs, and Enhance the General maintainability and dependability of your respective code.
Think Like a Detective
Debugging is not simply a technological task—it's a kind of investigation. To proficiently detect and repair bugs, developers need to tactic the procedure similar to a detective resolving a mystery. This state of mind aids stop working advanced challenges into workable pieces and follow clues logically to uncover the root trigger.
Commence by collecting evidence. Consider the indicators of the challenge: mistake messages, incorrect output, or effectiveness difficulties. Identical to a detective surveys against the law scene, obtain just as much suitable facts as you may devoid of leaping to conclusions. Use logs, take a look at scenarios, and person stories to piece jointly a clear image of what’s happening.
Next, form hypotheses. Ask yourself: What could be causing this actions? Have any variations a short while ago been built into the codebase? Has this difficulty transpired just before below similar instances? The target is usually to narrow down possibilities and establish likely culprits.
Then, check your theories systematically. Try to recreate the condition in the controlled ecosystem. When you suspect a particular function or part, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, ask your code issues and Allow the effects direct you nearer to the reality.
Fork out near attention to modest particulars. Bugs normally conceal in the minimum expected destinations—like a lacking semicolon, an off-by-1 error, or maybe a race problem. Be complete and individual, resisting the urge to patch The difficulty without having fully comprehension it. Temporary fixes may well hide the true problem, just for it to resurface afterwards.
Finally, retain notes on Everything you tried out and learned. Equally as detectives log their investigations, documenting your debugging system can preserve time for upcoming concerns and enable Other people fully grasp your reasoning.
By pondering just like a detective, builders can sharpen their analytical skills, technique complications methodically, and turn out to be simpler at uncovering concealed challenges in complicated programs.
Generate Tests
Creating assessments is among the simplest ways to boost your debugging techniques and In general development efficiency. Exams not merely support capture bugs early but will also function a security Web that offers you confidence when creating modifications in your codebase. A properly-examined application is simpler to debug since it lets you pinpoint particularly wherever and when a challenge happens.
Begin with device exams, which target particular person capabilities or modules. These small, isolated tests can rapidly reveal whether or not a specific bit of logic is Doing the job as envisioned. Any time a exam fails, you straight away know wherever to glance, drastically minimizing time invested debugging. Device assessments are Specially beneficial for catching regression bugs—problems that reappear after Beforehand staying mounted.
Subsequent, combine integration assessments and stop-to-finish checks into your workflow. These enable make certain that numerous aspects of your software function together efficiently. They’re specifically useful for catching bugs that come about in sophisticated systems with many factors or providers interacting. If something breaks, your assessments can tell you which part of the pipeline unsuccessful and under what ailments.
Creating checks also forces you to Imagine critically about your code. To check a function thoroughly, you will need to understand its inputs, predicted outputs, and edge cases. This amount of understanding In a natural way leads to higher code composition and fewer bugs.
When debugging a problem, crafting a failing check that reproduces the bug is often a powerful initial step. When the test fails constantly, you could concentrate on repairing the bug and check out your check move when The difficulty is resolved. This strategy makes sure that a similar bug doesn’t return in the future.
In a nutshell, producing checks turns debugging from a aggravating guessing match right into a structured and predictable process—aiding you catch additional bugs, a lot quicker and much more reliably.
Get Breaks
When debugging a tough difficulty, it’s easy to become immersed in the trouble—watching your display screen for several hours, hoping Alternative after Answer. But Just about the most underrated debugging equipment is actually stepping absent. Getting breaks can help you reset your head, lower irritation, and infrequently see The difficulty from the new point of view.
When you are way too near to the code for way too prolonged, cognitive tiredness sets in. You would possibly get started overlooking obvious mistakes or misreading code that you just wrote just hrs previously. In this particular condition, your brain gets to be much less efficient at problem-resolving. A brief stroll, a coffee break, and even switching to a distinct activity for 10–15 minutes can refresh your aim. Lots of builders report getting the basis of a difficulty once they've taken time to disconnect, letting their subconscious work during the qualifications.
Breaks also support stop burnout, especially all through extended debugging periods. Sitting before a display screen, mentally stuck, is don't just unproductive but in addition draining. Stepping away helps you to return with renewed Electricity as well as a clearer mindset. You may perhaps quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
In the event you’re trapped, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a 5–ten minute crack. Use that time to maneuver around, extend, or do something unrelated to code. It could feel counterintuitive, Specially under restricted deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, taking breaks just isn't an indication of weakness—it’s a wise tactic. It gives your brain Place to breathe, increases your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Every bug you experience is much more than simply A short lived setback—It is a chance to increase to be a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural challenge, every one can teach you some thing useful in case you go to the trouble to reflect and analyze what went Incorrect.
Commence by asking by yourself some vital questions once the bug is resolved: What brought on it? Why did it go unnoticed? Could it happen to be caught earlier with much better methods like unit testing, code critiques, or logging? The answers frequently reveal blind spots in your workflow or understanding and help you build stronger coding habits going ahead.
Documenting bugs can even be an outstanding routine. Hold a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and That which you figured out. After a while, you’ll start to see patterns—recurring challenges or popular issues—you can proactively prevent.
In crew environments, sharing Everything you've learned from the bug using your peers is usually In particular strong. Regardless of whether it’s through a Slack information, a short write-up, or A fast understanding-sharing session, helping Some others stay away from the same challenge boosts group performance and cultivates a more robust Studying society.
A lot more importantly, viewing bugs as classes shifts your frame of mind from aggravation to curiosity. In lieu of dreading bugs, you’ll start off appreciating them as essential portions of your improvement journey. In fact, a number of the best developers are usually not the ones who generate excellent code, but individuals that continually master from their blunders.
Eventually, Each and every bug you take care of adds a whole new layer to your ability established. So next time you squash a bug, take a minute to replicate—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging capabilities usually takes time, apply, and endurance — though the payoff is huge. It can make you a far more efficient, assured, and capable developer. The subsequent time you might be knee-deep in the mysterious bug, recall: debugging isn’t a chore — it’s an opportunity to become far better at That which you do.